An OPCode is the numeric identifier of a single operation that can be performed by the Zend Virtual Machine (Zend VM). After PHP has scanned the human-readable source code and chunked it into tokens, these tokens are grouped together in the parsing phase. The little expressions are in turn compiled or translated into opcodes, which are instructions for the Zend VM to execute as a unit. Opcodes end up in an array from where they are picked up one by one and run through the execution engine.
The list of OPCodes in PHP 7 is readily accessible. Better still, the PHP source code offers a closer insight. The macro definitions of opcodes are in Zend/zend_vm_opcodes.h
, where we see the constant names prefixed with "ZEND_".
static const char *zend_vm_opcodes_names[199] = {
"ZEND_NOP",
"ZEND_ADD",
"ZEND_SUB",
"ZEND_MUL",
"ZEND_DIV",
"ZEND_MOD",
}
For each Zend VM operation, there is a native C function to run, and it is given either 0, 1 or 2 operands. After completing the operation, it gives a result plus any additional information. Also, the operation holds information on the types of any operands passed in, the result type and the opcode.
The VLD extension allows us to see what the Zend engine is doing with the opcodes. This extension allows us to compare what happens when we run two similar scripts. For our experiment, we will look at string interpolation and concatenation.
<?php
$name = "Adele";
echo "$name is the best singer in the world.";
echo PHP_EOL;
<?php
$name = "Adele";
echo $name . ' is the best singer in the world.';
echo PHP_EOL;
Running these scripts with VLD on the command line gives the following output in PHP 7.1:
Test 1
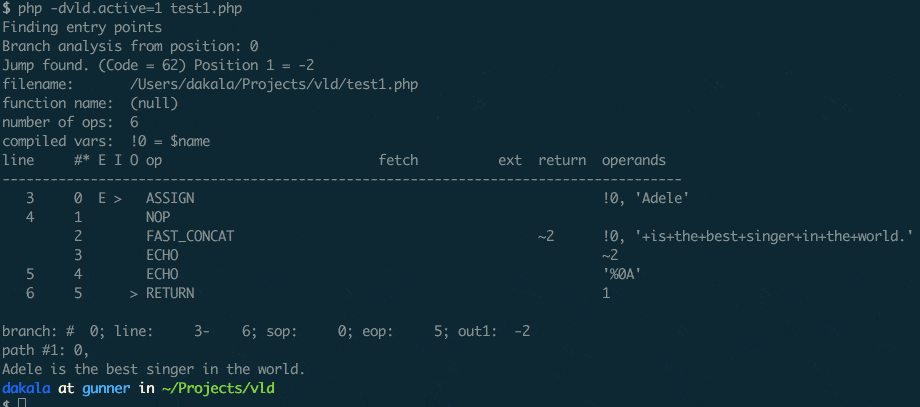
Test 2
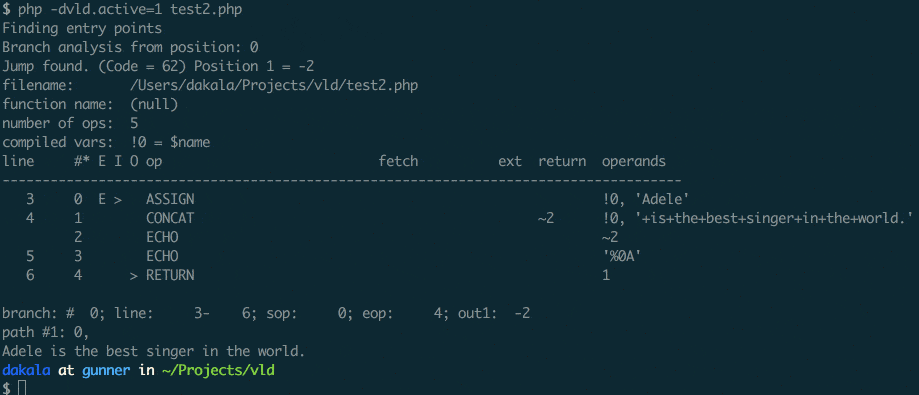
The script with string interpolation (test1.php
) takes 6 operations while the concatenation (test2.php
) has 5. The difference in operations comes from the NOP
and FAST_CONCAT
in the first one against CONCAT
in the second. Although they are similar, we can conclude that the Zend VM does more work when interpolating than concatenating strings.
If you are curious enough and able to get VLD working, you may run more interesting code snippets through this excellent extension and find out for yourself which option is less tasking for the PHP engine.