JavaScript continues to be the world’s most popular programming language. If you want to design anything on the web, chances are you’ll need JavaScript. The popularity of the language comes in part from the many JavaScript tools that make programming easy and enjoyable. This is a list with some of the most useful tools for JavaScript.
- IDEs and Editors
- Task Runners and Module Bundlers
- Documentation Software
- Testing Frameworks
- Linting Software
- Debuggers
- Security Analyzers
- Package Managers
IDEs and Editors
It matters where you write your code. Where JavaScript editors offer smooth and responsive performance, JavaScript IDEs are generally used for more complex projects. They have more features, such as debugging functionality, support for ALM systems, and version control integrations.
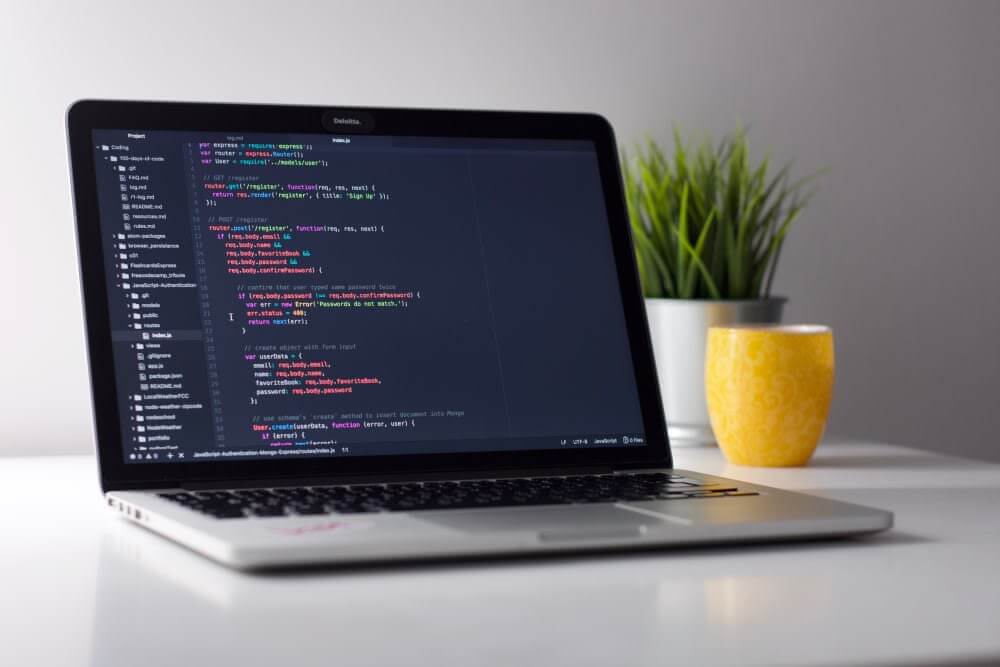
- Visual Studio Code: Microsoft’s well-known and beloved code editor. Visual Studio Code is the most popular development environment for pretty much any type of developer. It includes support for debugging, Git control, syntax highlighting, code refactoring, and much more.
- Sublime Text: a highly customizable, cross-platform code editor. Sublime Text sits somewhere in between a fully fledged IDE and a command line editor. Despite its many features, it’s one of the fastest code editors on this list. You can install 3rd-party plugins through its package manager called Package Control.
- Atom: a text editor written by the developers over at GitHub. Atom comes with plenty of features out of the box: cross-platform editing, a built-in package manager, smart autocompletion, find and replace, etc. There are also plenty of themes to customize its look and feel to your heart’s desires.
- WebStorm: an IDE tailored specifically for JavaScript. Made by JetBrains, WebStorm comes with features such as intelligent code completion, on-the-fly error detection, JavaScript navigation and refactoring. It also provides support for TypeScript, stylesheet languages, and the most popular JavaScript frameworks.
Task Runners and Module Bundlers
A few years ago, it would have been a waste of time to learn how to use JavaScript task runners and module bundlers. But JavaScript web apps and projects have become much more complex, to the point where task runners and module bundlers have become necessary components in a front-end developer’s workflow.
JavaScript task runners and module bundlers help with boring tasks such as minification, unit testing, and web page refreshing. While some of the below tools have a steep learning curve, they can save a developer plenty of time by automating many of the more menial programming tasks.
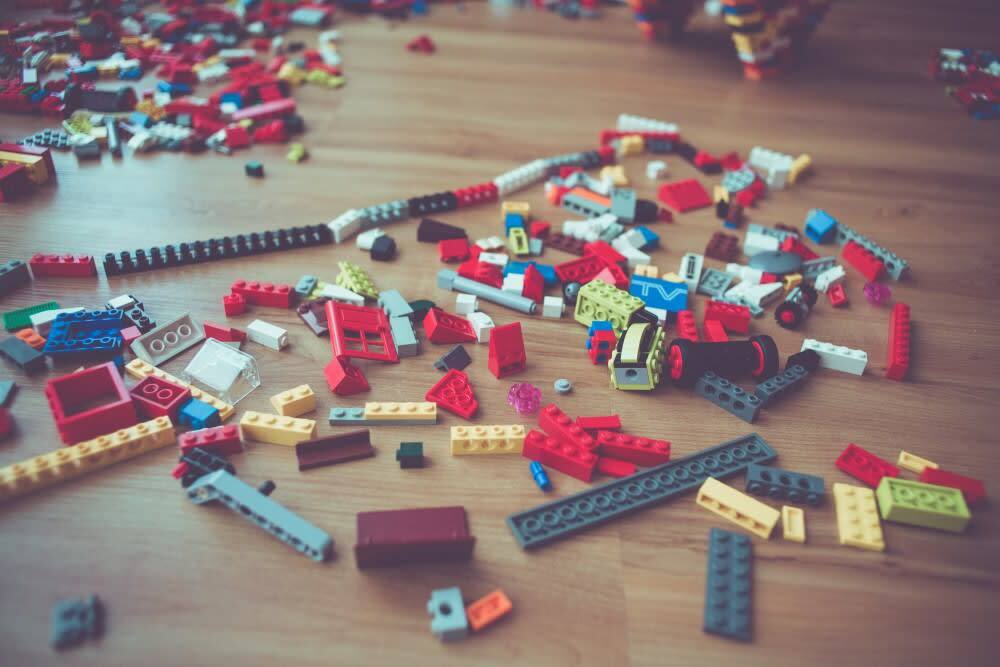
- esbuild: an extremely fast JavaScript bundler that doesn't require a cache. Different from other bundlers, esbuild is written in Go, which is why it's so fast. Has robust support for common JS modules.
- Gulp: defines and runs time-consuming tasks. Different from Grunt, Gulp defines tasks as JavaScript functions instead of configuration objects.
- Webpack: software that bundles all your JavaScript apps, as well different assets like images, font, and stylesheets. Webpack supports ESM and CommonJS.
- Rollup: a JavaScript module bundler that compiles small pieces of code into something larger and more complex, such as a library or application. Rollup uses the new standardized code format for code modules included in JavaScript’s ES6 revision.
- Parcel: a JavaScript module bundler that enables multi-core compilations for extremely fast performance. Also support CSS, HTML, and file assets out of the box.
Documentation Software
Software without documentation is a developer’s nightmare, but software with poorly written documentation is arguably even worse. It’s tempting to consider software documentation as an afterthought. The code will speak for itself, won't it? Spoiler alert: It won't.
Writing documentation is a complex, time-consuming, and often boring process. Software documentation tools ease some of that burden by automating a large part of the process. No longer should you skimp on writing documentation, because these tools make the job significantly easier.
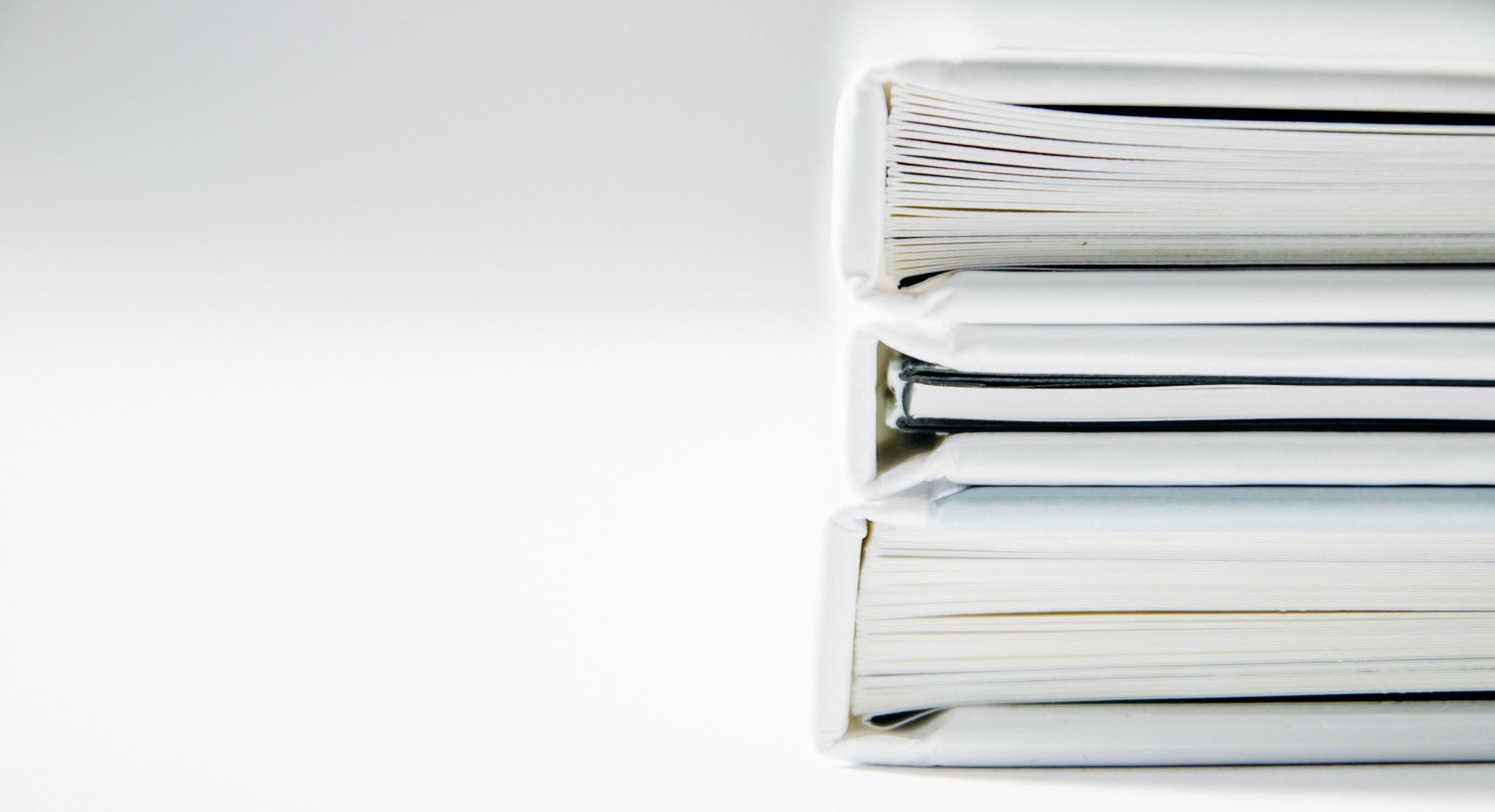
- Docco: a documentation generator written in Literate CoffeeScript. Docco generates an HTML document that shows your comments along with your code. All your comments are passed through Markdown while the code is passed through
Highlight.js
syntax highlighting. - Swagger: a great tool across the entire API lifecycle, from design to documentation. Swagger uses a set of rules and tools for describing APIs. It’s language-agnostic and readable for both humans and machines.
- YUIDoc: a Node app that generates API docs from comments in the source code. YUIDoc supports a wide range of JavaScript coding styles. Its output is API docs formatted as HTML pages.
- JSDoc: a markup language used to annotate JavaScript source code files, which it then uses to produce documentation in formats like HTML and RTF.
Testing Frameworks
Testing is an important part of the development process. You have to figure out if your code works under various circumstances. How else will you identify errors, gaps, or missing requirements?
JavaScript testing tools come in handy because of the growing complexity of JavaScript apps. Manual tests just aren’t feasible anymore. Use these testing tools to run a script and generate results automatically instead.
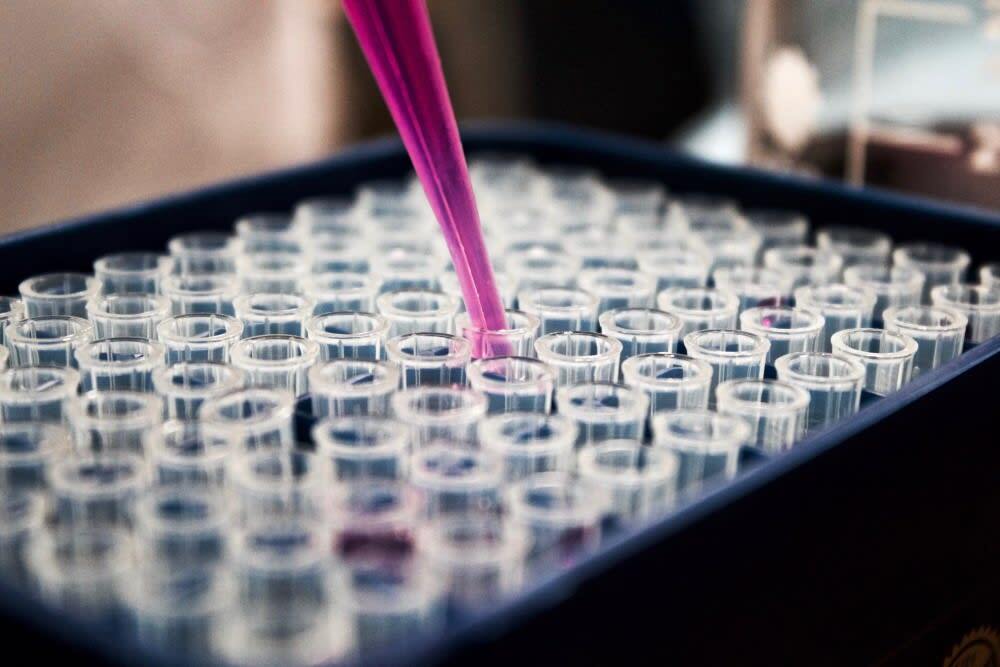
- Testing Library: a complete set of testing tools that encourage good testing practices. The testing tools developers were most satisfied with in the State of JavaScript 2021 survey.
- Vitest: a really fast unit-test framework powered by Vite, a rising star in the JavaScript ecosystem. Still under development, so be careful migrating your current testing setups, but already well-loved by many developers.
- Playwright: end-to-end testing for modern web apps. Supports all modern rendering engines, from Chromium to WebKit. Works cross-platform and cross-language (can be used for Python, .NET, and Java along with JavaScript and TypeScript).
- Cypress: an end-to-end JavaScript testing framework. Cypress takes snapshots as your tests run, automatically reloads whenever you make changes to your tests, and automatically waits for commands and assertions before moving on.
- Jest: a JavaScript testing framework that focuses on simplicity. Jest is used by Facebook to test all their JavaScript code (including their React apps). It has been built to work out of the box, without configuration, on most JavaScript projects.
Linting Software
Technically speaking, linting is part of testing. But it’s so important that it deserves a category of its own. Linting means running a program that checks your code for stylistic and programmatic errors.
Every front-end developer should use JavaScript linting tools to maintain the quality of their code. They improve the readability of your code, identify structural problems, find ugly syntax errors, and generally discover problems in your JavaScript code without you having to execute it.
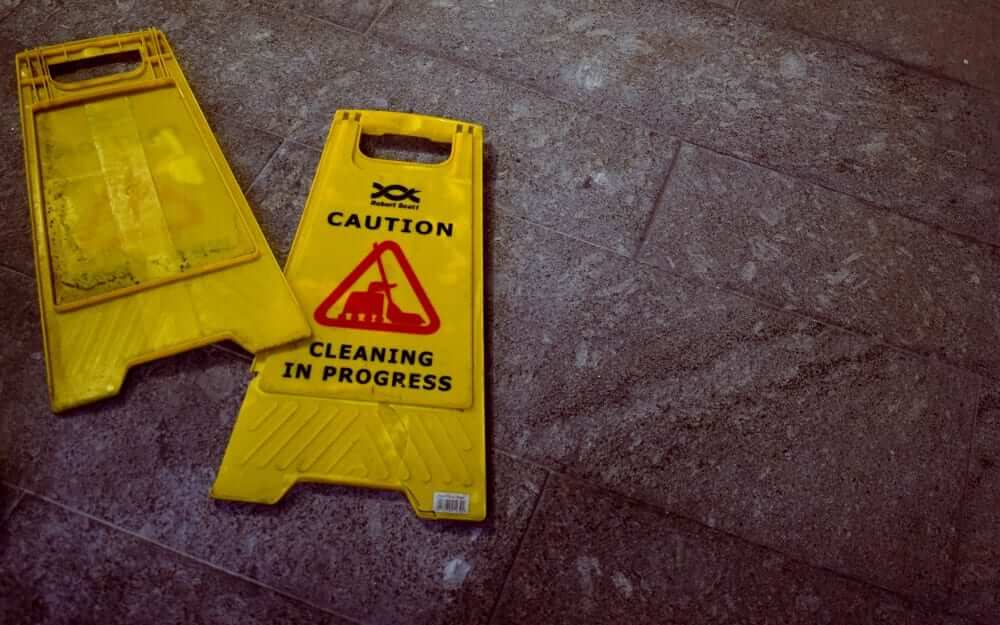
- ESLint: a favorite of many developers. ESLint is an open-source plugin for JavaScript and JSX that’s mainly used on the command line. All its rules are pluggable, so developers can create the linting rules they prefer.
- Flow: a static JavaScript code checked developed by Facebook. Flow checks your code for errors through static type annotations, but it only needs a minimum of such descriptions because it infers types and tracks data as it moves through your code.
- Prettier: an opinionated code formatter that saves you time discussing style in code reviews. Prettier integrates with most editors and supports JavaScript, HTML, CSS, GraphQL, Markdown, YAML, and more languages through community plugins.
Debuggers
Who doesn’t love finding and fixing bugs in code? That single comma you’ve misplaced in seven hundred lines of code? The illogical if-then statement that throws your machine into an infinite loop? The endless Googling for a solution? Oh, the joy.
JavaScript debugging tools make debugging a less frustrating, time-consuming, and laborious process. They are indispensable for figuring out where a little mistake snuck into your (otherwise impeccable) code.
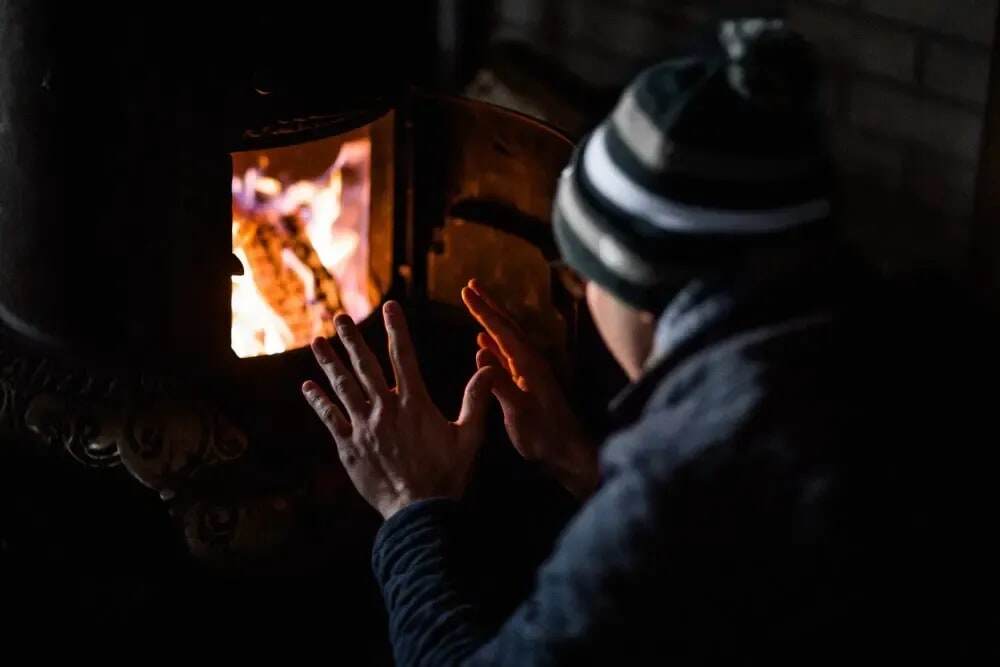
- Chrome DevTools: A set of tools built directly into Chrome. Chrome DevTools have multiple utilities that help debug your JavaScript code step by step.
- The Firefox JavaScript Debugger: developed by Mozilla, this JavaScript debugger can debug code run locally in Firefox or run remotely, for example on an Android drive running Firefox for Android.
- JavaScript Development Tools (JSDT): JSDT Debug helps you debug JavaScript using Rhino and Crossfire. Can debug JavaScript in all major browsers.
Security Analyzers
Cybersecurity has become a top priority for companies and countries. Vulnerable software is now a common attack vector for hackers. As a developer, the security of anything you code should be a priority.
JavaScript security analyzers look into your software’s dependencies and mitigate some of the security risk that comes with relying on other people’s plugins and frameworks.
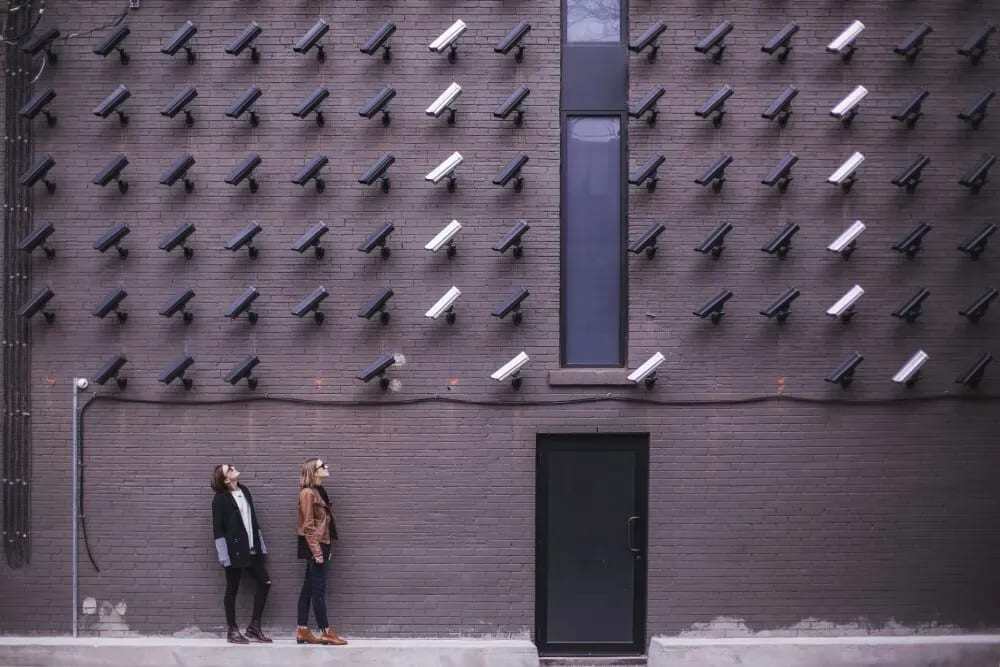
- Snyk: a developer-first application security analyzer used by millions of developers and companies such as Salesforce, Google, Microsoft, ASOS, and others. Snyk finds and fixes vulnerabilities for npm, Maven, NuGet, RubyGems, PyPi, and more.
- Retire.js: helps detect if you have versions of dependencies that are known to have security vulnerabilities. It's a command line scanner, has a Grunt plugin, a Chrome/Firefox extension, and a Burp/OWASP Zap plugin.
- Dependency-Check: attempts to detect publicly disclosed vulnerabilities contained within a project’s dependencies. Dependency-Check has a command line interface, a Maven plugin, an Ant task, and a Jenkins plugin.
- Acunetix: an application security testing platform for securing your websites, web apps, and APIs. Acunetix uses both black box and gray box hacking techniques to find vulnerabilities.
- OSS Index: a catalogue of open-source components and scanning tools to help developers identify vulnerabilities, understand risk, and keep their software safe. OSS Index supports multiple ecosystems, such as npm, Bower, Drupal, NuGet, and more.
Package Managers
A package manager is software that automatically installs, upgrades, configures, and removes the packages maintained in repositories. It also looks up your dependencies and ensures new versions of packages don’t break your code.
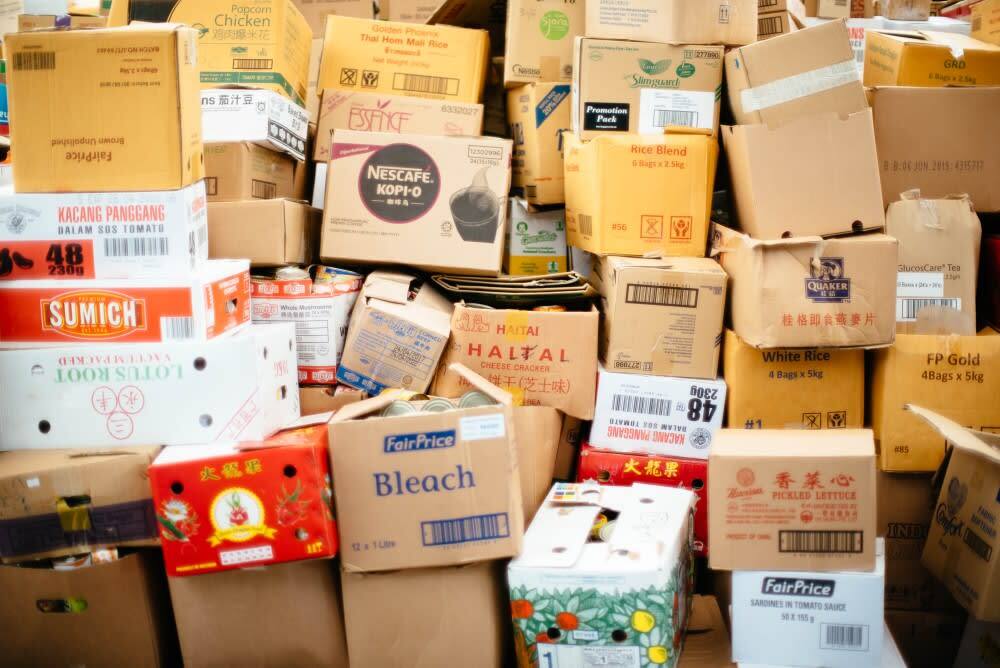
- npm: the center of JavaScript code sharing. More than eleven million developers use npm. Over the years, the JavaScript package manager has grown into the largest software registry in the world, with more than one million packages available.
- Yarn: the biggest competitor of npm. Developed by Facebook and released in 2016, Yarn addressed some of the biggest shortcomings of npm at that time (although npm has caught up considerably since then).
This was a list of 31 JavaScript tools that can make the life of any JavaScript developer significantly easier. We hope you have found at least one new tool that will turn you into a more productive developer.